- Get link
- X
- Other Apps
LINQ is a powerful feature in C# that provides a convenient way to query data from various sources. When combined with Entity Framework Core (EF Core), LINQ becomes a key tool for interacting with databases. However, optimizing LINQ queries is crucial for achieving peak performance in data retrieval and manipulation. In this article, we’ll explore 10 essential tips to boost the performance of LINQ queries using EF Core.
1 — Use AsNoTracking for Read-Only Operation

This helps improve performance for read only operations by skipping the change tracking process.
2 — Avoid Selecting Unnecessary Columns

Only select the columns that you need, as selecting unnecessary columns can impact query performance
3 — Use FirstOrDefault Instead of SingleOrDefault
When Possible:

FirstOrDefault is often more performant than SingleOrDefault, especially when dealing with large datasets.
4 — Be Mindful of Eager Loading:
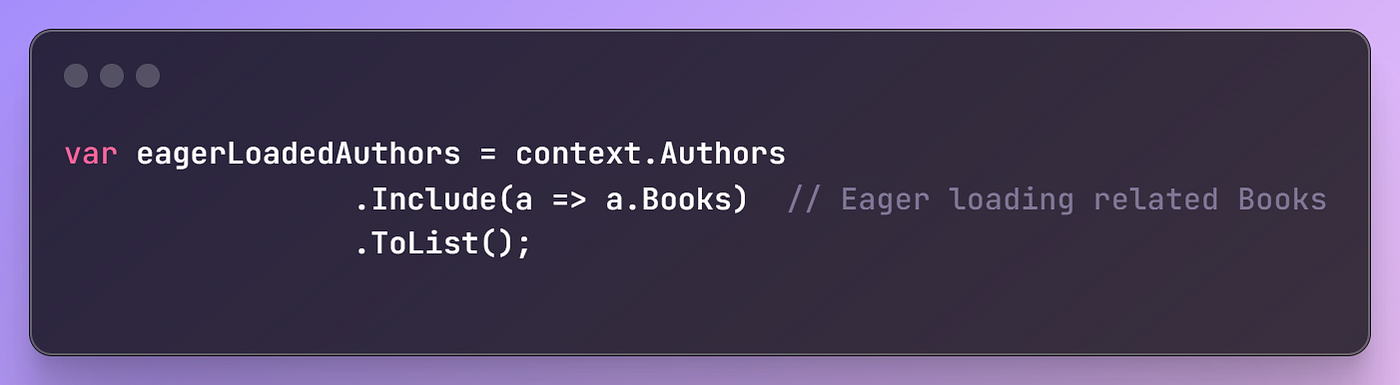
Use Include to eagerly load related entities only when necessary to avoid unnecessary data retrieval.
5 — Avoid N+1 Query Issue with Load or Include:
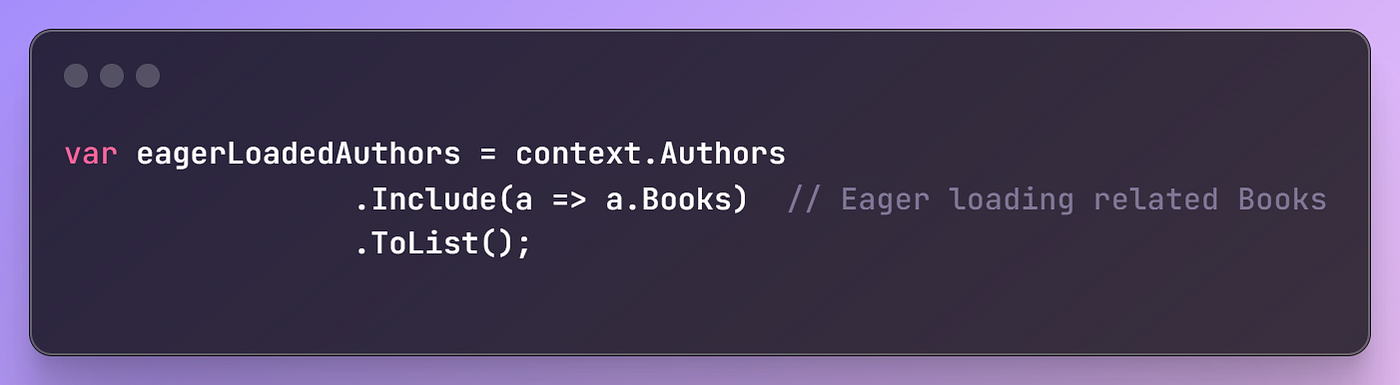
Use Include or Load to retrieve related entities in a single query, preventing the N+1 query problem.
6 — Batching Updates and Inserts:

When dealing with bulk updates or inserts, consider using batching to reduce round trips to the database.
7 — Use Compiled Queries:

Compiled queries can provide a performance boost by pre-compiling the LINQ query.
8 — Use ToList Wisely:
Avoid premature materialization of queries by deferring the use of ToList until the last moment in your code execution.
9 — Use Take and Skip for Paging:
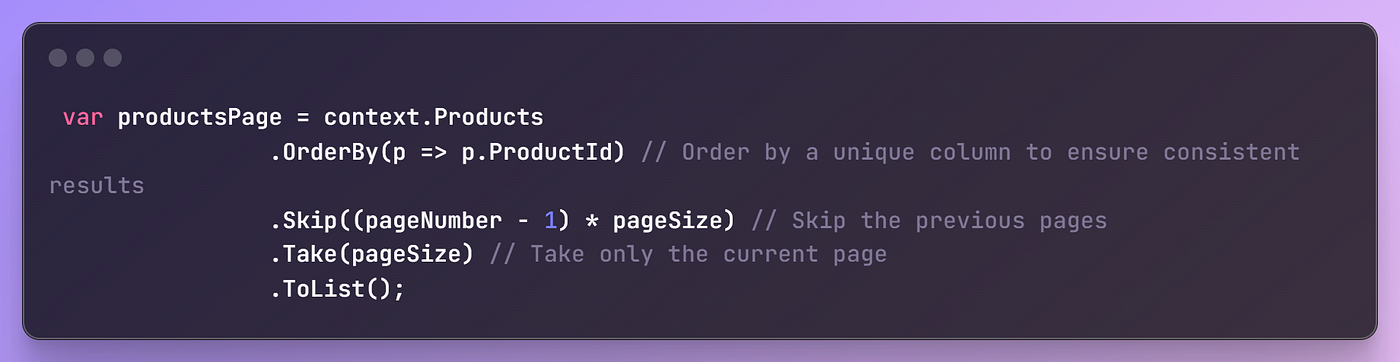
10 — Raw SQL Queries for Complex Operations:
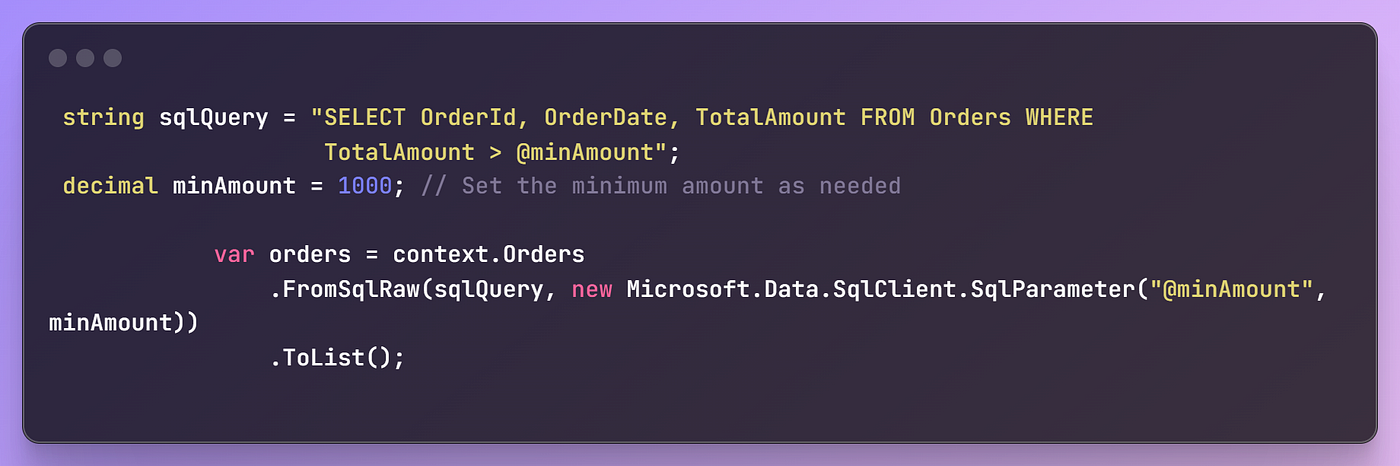
For complex queries that are hard to express using LINQ, consider using raw SQL queries.
- Get link
- X
- Other Apps
Comments